ReactJS Tutorial for Beginners
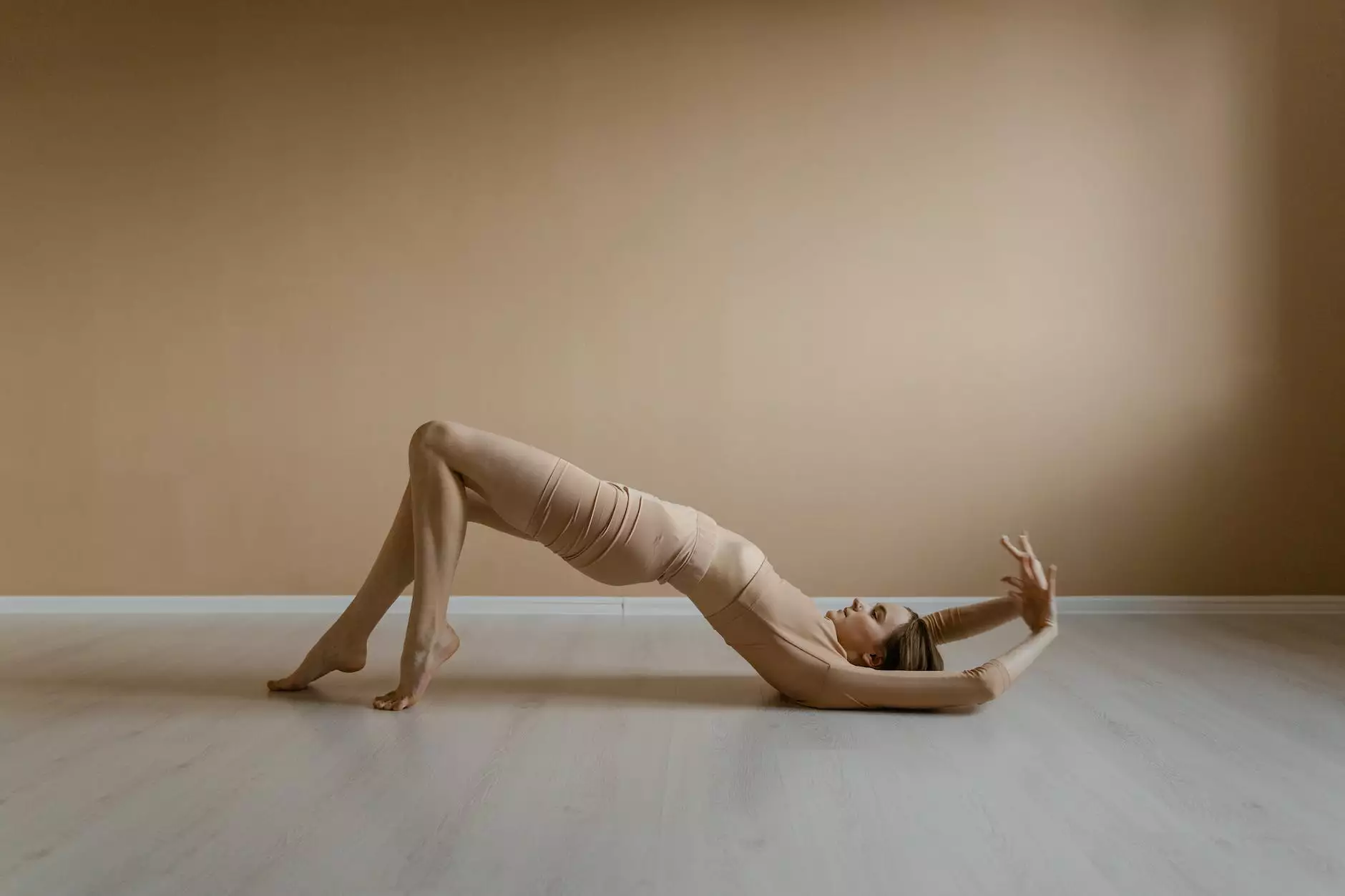
Welcome to the ReactJS Tutorial for Beginners, brought to you by CI Advertising. In this comprehensive tutorial, we will guide you through the fundamentals of ReactJS, ensuring that you gain a solid understanding of this powerful JavaScript library.
What is ReactJS?
ReactJS is an open-source JavaScript library designed for building user interfaces. It was developed by Facebook and has gained popularity due to its efficiency, reusability, and component-based approach. With ReactJS, developers can easily create interactive and dynamic web applications.
Why Learn ReactJS?
Learning ReactJS opens up a plethora of opportunities in the field of web development. As one of the most in-demand skills, mastering ReactJS can enhance your career prospects and enable you to build modern, responsive, and fast web applications. Whether you are a beginner or an experienced developer, ReactJS is a valuable addition to your skill set.
Getting Started
In this section, we will guide you through the initial steps of setting up your development environment to start coding in ReactJS.
Step 1: Install Node.js and npm
To develop ReactJS applications, you need to have Node.js and npm (Node Package Manager) installed on your machine. Node.js allows you to run JavaScript on the server-side, while npm helps with managing packages and dependencies. Visit the official Node.js website to download and install the latest version for your operating system.
Step 2: Create a New React Project
Once you have Node.js and npm installed, open a terminal or command prompt and run the following command to create a new React project:
npx create-react-app my-react-appThis command will generate a new React project in a directory called "my-react-app".
React Components
ReactJS is centered around the concept of components. Components are the building blocks of React applications and can be thought of as reusable UI elements. Each component encapsulates its own functionality and can contain a combination of HTML-like syntax and JavaScript logic.
Here's an example of a simple component:
import React from 'react'; class HelloWorld extends React.Component { render() { return ; } } export default HelloWorld;In this example, we create a component called HelloWorld that renders a simple heading element with the text "Hello, World!". Components can be composed and nested to build complex user interfaces.
Working with State and Props
One of the key features of ReactJS is the ability to work with state and props. State represents the internal data of a component, while props are used to pass data from a parent component to its child components.
Here's an example of a component that utilizes both state and props:
import React from 'react'; class Counter extends React.Component { constructor(props) { super(props); this.state = { count: 0 }; } incrementCount() { this.setState(prevState => ({ count: prevState.count + 1 })); } render() { return ({this.props.title}
Count: {this.state.count}
this.incrementCount()}>Increment ); } } export default Counter;In this example, we create a Counter component that displays a count value and a button to increment it. The count value is stored in the component's state, and the title is passed as a prop from the parent component.
Conclusion
Congratulations! You have now completed our ReactJS Tutorial for Beginners. We hope this tutorial has provided you with a solid foundation in ReactJS development. Remember to practice regularly and explore more advanced concepts to further enhance your skills.
By mastering ReactJS, you are well-equipped to build modern and interactive web applications. Stay curious, keep learning, and leverage your newfound knowledge to unlock exciting opportunities in the world of web development!
© 2021 CI Advertising | Business and Consumer Services - Marketing and Advertising